Coding Good Habits
Code Style
This page describes the best practices to follow in order to create Eggplant Functional scripts.
Script Header
At the beginning of any script/snippet, a header should be attached to give a clear overview of the script. Configuration control details can also be placed in this header portion to follow good script versioning control practices. It should list the script name, parameter/returned values list, author, version and change history of the script.
(**
UserData - Populates the new user data fields
@Params Username - String Describing Username
@Returns SaveFlag - Boolean for the Form Save Flag
@Version - 0.2 09/02/2022
@ChangeReason - AUB-11098 Review - Added Assertion
**)
Naming Conventions
For consistency and due to the fact that Eggplant Functional does not allow white spaces within names in scripts, the naming of function and variable names should be in camelCase. This aids the readability of the code for others and provides a consistent approach to coding the scripts.
The naming of a variable (local, global and universal variable) should be brief and descriptive as this will allow easy reading when revisiting code. The use of single ambiguous letters should be avoided.
Images should be saved with reference to the application and page/screen within the application that they belong to. Images should be saved descriptively to allow developers to easily check if an image is already available to avoid duplication.
// Function to launch browser
to function_a b, c
// open run dialogue
typetext windowskey, "r"
wait 1
// type in browser and url
typetext b && c, returnkey
// wait for browser logo
waitfor 20, image:"image1"
end function_a
// Function to launch browser
to launchBrowser browser, url
// open run dialogue
typetext windowskey, "r"
wait 1
// type in browser and url
typetext browser && url, returnkey
// wait for browser logo
waitfor 20, image:"logos/browsers/" & browser & "/Home"
end launchBrowser
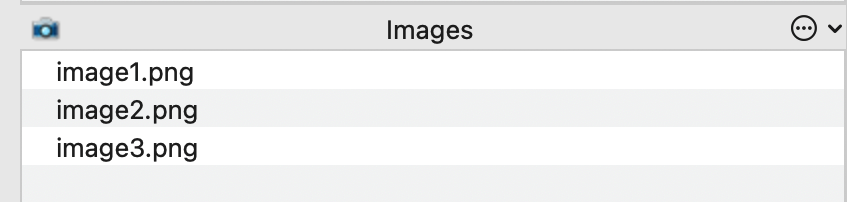
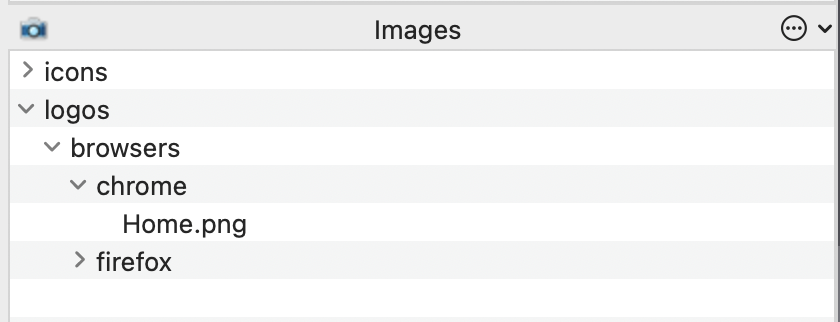
Commenting
Comments are to be included throughout the script to aid the readability of the code. In general, each function in a script should include comments detailing the flow/structure of the function. The comment block should be in the format:
// Comment Detail
Any complex data structures used should have a definition of content if the naming of the data elements do not make it self-evident.
Compare the two blocks of identical code below:
// Create Results file and open it
put formattedTime("%d%m%Y_%H%M") into timestamp
copy file ResourcePath("EggplantSampleData.xlsx") as ResourcePath("ResultsData_"×tamp&".xlsx")
set spreadsheet to Workbook(ResourcePath("ResultsData_"×tamp&".xlsx"))
set sheetData to spreadsheet.Worksheet("Sheet1")
// Calculate timestamp to use in results filename
put formattedTime("%d%m%Y_%H%M") into timestamp
// Copy the results file template and rename it using the timestamp
copy file ResourcePath("EggplantSampleData.xlsx") as ResourcePath("ResultsData_"×tamp&".xlsx")
// Open spreadsheet to create new headers user for the results file
set spreadsheet to Workbook(ResourcePath("ResultsData_"×tamp&".xlsx"))
// set the tab
set sheetData to spreadsheet.Worksheet("Sheet1")
Where handlers within the script are present, then the following comment block should be placed before the handler, with input and output parameters (if any) are described:
(**
Handler Name - Handler Description
@Params ParameterName - Parameter Type and Description
@Returns ReturnResult - Result Type and Description
**)